Macro scripts
Macros are one-file C# files which can be executed within for HaxeDevelop.
Usage
Here is a macro which "flattens" single line XML code in the current document.
// FlattenXML.cs
using System;
using PluginCore;
public class FlattenXML
{
public static void Execute()
{
ScintillaNet.ScintillaControl sci = PluginBase.MainForm.CurrentDocument.SciControl;
if (sci == null) return; // document not editable
string src = sci.Text; // WARNING: reading/writing sci.Text property is slow
sci.Text = src.Replace("><", ">\n<");
}
}
Save the script
We recommend to save this as .cs file in your user directory (from HaxeDevelop main menu: Tools > User Config Files) which corresponds to the $(BaseDir)
variable, but it can really be anywhere.
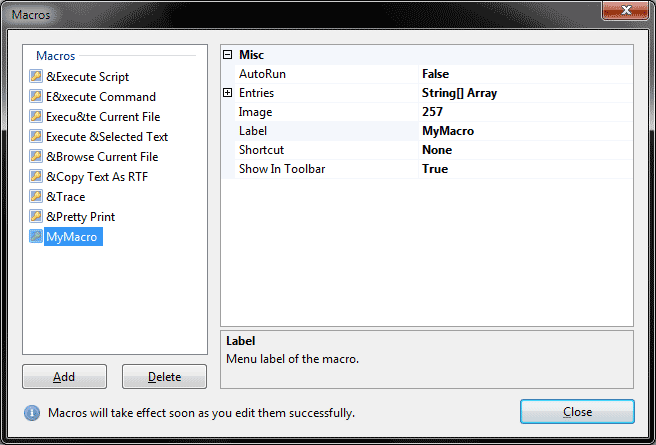
Add the script
The macro properties are pretty straightforward:
- AutoRun - Optionally execute macro on startup
- Entries - List of basic macro commands (click
<...>
to edit) - Image - Pick an icon number
- Label - Text of the menu item
- Shortcut - Obviously a shortcut to execute your macro
Test the script
For quick testing you can simply select your .cs file manually from main menu: Macros > Run Script.
During development, use this action to make sure the script is reloaded/recompiled every time:
ExecuteScript|Development;$(BaseDir)\FlattenXML.cs
When your script is completed, you want to make sure the script is only compiled once:
ExecuteScript|Internal;$(BaseDir)\FlattenXML.cs
Note: Please don't confuse macro scripts with the Haxe Macros, this is something different.
Snippets editor
The Snippet Compiler Live 2008 Ultimate Edition for Developers!
This is free, lightweight C# editor offering actually good code completion and compilation - precisely designed to quickly code and compile one class (typically to create a little command line application).
So download Snippet Compiler (SC) and run it. You'll be presented with a default C# class for a command line application.
The template class has 2 methods: RunSnippet and Main. This is a nice way to let you write your code in RunSnippet and to not worry about the usual try/catch and ReadKey when you test a command-line application.
For HaxeDevelop macro scripts:
- Compile your script using SC to trap compile errors.
- this means a
public static void Main()
method is required, SC compiles command line application only - Keep the default class as-is. Use
RunSnippet()
for your tests outside HaxeDevelop.
- For most code completion needs you'll have to add a "reference" to FlashDevelop's PluginCore:
- In SC, select: Tools > References > File System
- Choose
<...>
to browse to HD program files and choose PluginCore.dll. Double-click once to add it in the DLL list at the bottom of the dialog, then click Ok. You may have to re-add the reference when you restart SC.
- You will then simply add a
public static void Execute()
method with your FD script. - Code, compile with Ctrl+Shift+B to control your code is ok, then test in HaxeDevelop.